Notes on NextJS: Routing in NextJS
Over the last few weeks, I’ve been delving deeper into Next.js, the React meta-framework. One of the initial hurdles when acquainting oneself with a new framework is understanding its routing mechanism, a fundamental feature of any web application.
Next.js takes a unique folder-based approach, distinguishing itself from other frameworks I’ve worked with. Unlike Angular and Vue, which utilise a component-based router system detached from the application’s source folder structure, Next.js integrates routing seamlessly into its architecture, building upon React’s inherent lack of a default routing system. It’s fascinating to observe how Vercel has seamlessly integrated this functionality into the Next.js framework.
The folder-based routing system in Next.js is remarkably straightforward. Each route within your application corresponds to a sub-folder in your source directory, with each sub-folder containing a page.tsx
file representing the destination of that route.
While this approach is innovative, it’s not entirely novel. In the early days of web development, before the advent of JavaScript frameworks and server-side rendering, we employed a similar folder-based routing system, where each route corresponded to an index.html
file within its respective folder. For example, the homepage’s route would be http://www.mysite.com/index.html
, while a separate route would be http://www.mysite.com/news/index.html
. This historical perspective underscores the cyclical nature of ideas in the web industry.
It’s heartening to witness the simplification of routing, particularly with Next.js’s support for dynamic routes. Moreover, it’s intriguing to note that other frameworks, such as Nuxt for Vue and AngularJS for Angular, are also adopting folder-based approaches. This resurgence of an old concept underscores the enduring nature of certain ideas within the ever-evolving landscape of web development.
Demo app
https://github.com/Stephenradams/star-wars-search
To learn a bit about the routing and how to call external APIs in Next I created a small demo app using the Star Wars API to get lists of Films, People and Planets. Its a very simple app, but these small demo apps are a great way of learning new concepts.
In this demo app you can see that the folder structure uses a folder for each sub-section (films, planets, species, starships):
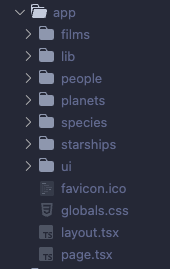
Inside each folder is a page.tsx
file which is the ‘homepage’ of the route, these sub-folders can also have a layout.tsx
file which defines the layout of the page, by overriding the main layout.tsx
layout.
To support dynamic routes, NextJS uses a special sub-folder named [id]
where the dynamic property, in this case an id
number used to direct the user to another page.tsx
file inside this special sub-folder:
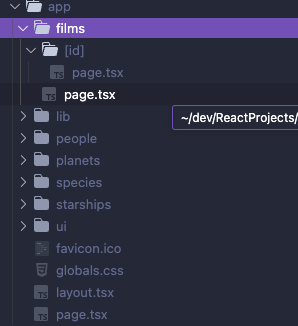
This dynamic parameter is accessible within the page.tsx
file via the component params:
export default async function Page({ params }: { params: { id: string } }) {
const filmId = params.id;
const filmDetails: FilmDetails = await getFilm(params.id);
console.log(filmDetails.title);
So in this example code I’m getting the ID from the params and then passing it to my API call. It’s so simple and straight forward, I’m not adding anything to a routes file, or setting up routes and getting the URL params is extremely easy.
I really like the approach Next has taken with routing, now I’m going to take a look at handling forms and form data which is another common function of web apps.